Breaking the Cycle: Solving "Too Many Redirects" in Android WebView for a Seamless User Experience
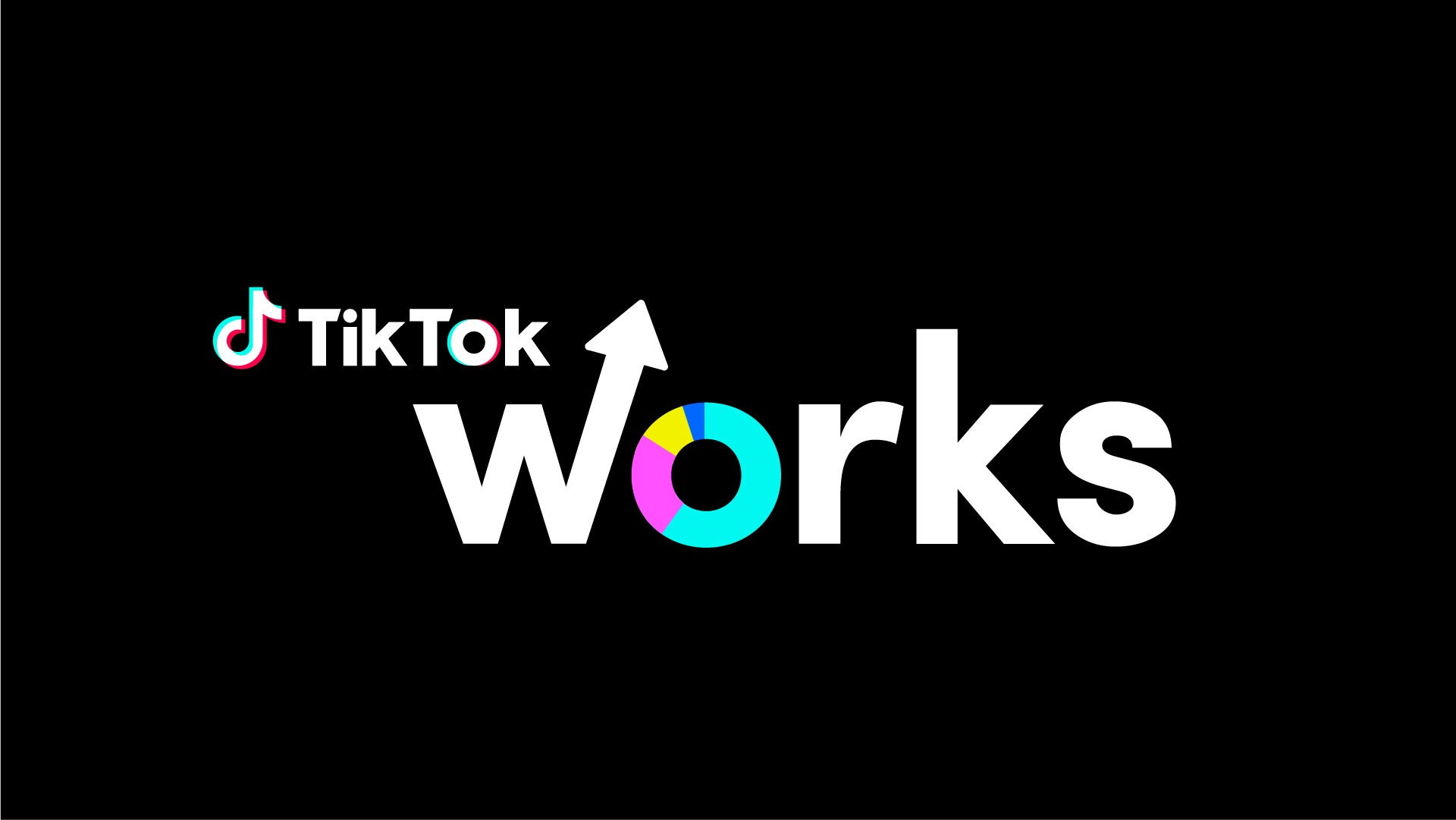
Let’s dive into the heart of the issue. Why does the "Too Many Redirects" error occur in Android WebView? The answer is rooted in how WebView handles HTTP redirects. When a URL within a WebView redirects to another URL, and this process continues, it can create an infinite loop, causing the WebView to throw a "Too Many Redirects" error. Essentially, WebView gets caught in a loop where it’s redirected too many times between different URLs, and it doesn't know how to escape.
Understanding HTTP Redirects:
HTTP redirects are instructions that tell a browser to fetch a different URL from the one requested. There are different types of HTTP redirects, including 301 (permanent redirect) and 302 (temporary redirect). These are commonly used to forward users to a new page when a URL changes or to manage load balancing by redirecting traffic.
The Role of WebView:
In Android, WebView is a system component powered by Chrome that allows Android apps to display web content. It’s a powerful tool but also comes with its quirks. One of these quirks is its handling of redirects. Unlike a standard browser, WebView might not always follow redirects as expected, especially when the redirects are too complex or nested.
Common Causes of "Too Many Redirects":
- Misconfigured Server Settings: Sometimes, the server hosting the web content is not configured correctly, causing it to send multiple redirects in a loop.
- Improper URL Handling: This could involve incorrect handling of cookies or session data that confuses WebView, leading to repeated redirects.
- Infinite Redirect Loops: Sometimes, a URL redirects to another URL, which eventually redirects back to the original URL, creating an infinite loop.
- Cross-Domain Redirects: Redirects involving multiple domains can cause confusion in WebView, particularly if there are restrictions or mismatches in the way these domains handle cookies and sessions.
- Cached Data: WebView may use cached data that no longer aligns with the server’s current state, leading to unexpected redirects.
Why Should You Care?
The impact of the "Too Many Redirects" error is significant. For developers, it can result in:
- Poor User Experience: Users might get stuck in a loop, unable to reach the intended content, leading to frustration.
- Increased App Uninstalls: Frustrated users are more likely to uninstall an app, especially if they encounter persistent issues.
- Negative Reviews: Persistent issues lead to negative reviews in app stores, which can deter potential users.
- Reduced Revenue: For apps that rely on ad impressions or in-app purchases, every frustrated user represents lost potential revenue.
Diagnosing the Problem:
Before you can fix the issue, you need to diagnose it. Here’s how:
- Use WebView Debugging Tools: Android Studio provides tools for debugging WebView, including the ability to see the network requests and responses. This can help you identify where the redirect loop starts.
- Check the Server Configuration: Make sure that the server hosting the content is correctly configured, with appropriate redirect rules.
- Inspect URL Handling: Ensure that the app correctly handles URLs, cookies, and session data to avoid unnecessary redirects.
Fixing the "Too Many Redirects" Error:
Once you’ve identified the cause, you can take steps to fix the problem:
Handle Redirects in Code: You can intercept URL requests in WebView and manage redirects manually. This allows you to control the flow and prevent infinite loops.
javawebView.setWebViewClient(new WebViewClient() { @Override public boolean shouldOverrideUrlLoading(WebView view, String url) { if (url != null && url.startsWith("http")) { view.loadUrl(url); return true; } return false; } });
Update WebView Settings: Adjust the WebView settings to handle redirects more gracefully. For example, enabling or disabling certain features can help manage how WebView processes redirects.
javaWebSettings webSettings = webView.getSettings(); webSettings.setJavaScriptEnabled(true); webSettings.setDomStorageEnabled(true);
Clear WebView Cache: Clearing the cache ensures that outdated data doesn’t interfere with the current session.
javawebView.clearCache(true);
Use SSL Certificates Correctly: Ensure that all SSL certificates are properly configured, especially if dealing with multiple domains. Misconfigured SSL can lead to redirects and loops.
javawebView.setWebViewClient(new WebViewClient() { @Override public void onReceivedSslError(WebView view, SslErrorHandler handler, SslError error) { handler.proceed(); // Ignore SSL certificate errors } });
Limit the Number of Redirects: You can implement a limit on the number of redirects that WebView will follow. This can prevent infinite loops and allow you to catch potential errors.
javaprivate int redirectCount = 0; private final int MAX_REDIRECTS = 5; webView.setWebViewClient(new WebViewClient() { @Override public boolean shouldOverrideUrlLoading(WebView view, String url) { redirectCount++; if (redirectCount < MAX_REDIRECTS) { view.loadUrl(url); return true; } return false; } });
Test Across Different Scenarios: Make sure to test the WebView implementation across various scenarios, including different devices, Android versions, and network conditions, to ensure robustness.
The Business Case for Fixing the Error:
Fixing the "Too Many Redirects" error isn’t just about improving the technical aspects of your app; it’s about safeguarding your business. Here’s why:
- User Retention: A smooth, error-free user experience leads to higher retention rates. Users are more likely to stay engaged with an app that doesn’t frustrate them.
- Revenue Protection: For apps that monetize through ads or in-app purchases, every user counts. Fixing this issue ensures that users stay within the app longer, increasing the chances of revenue generation.
- Brand Reputation: Consistently positive user experiences lead to better reviews, which can enhance your app’s visibility and credibility in the app store.
Conclusion:
The "Too Many Redirects" error in Android WebView is more than just a technical nuisance—it’s a critical issue that can impact user experience, revenue, and brand reputation. By understanding the root causes and implementing strategic fixes, you can turn a potentially frustrating experience into a seamless one. Your users deserve a smooth, intuitive experience, and with the right approach, you can ensure that they get it.
Now that you’re armed with the knowledge to fix this issue, take the time to implement these solutions in your app. The payoff will be worth it—a more robust app, happier users, and a healthier bottom line.
Top Comments
No Comments Yet